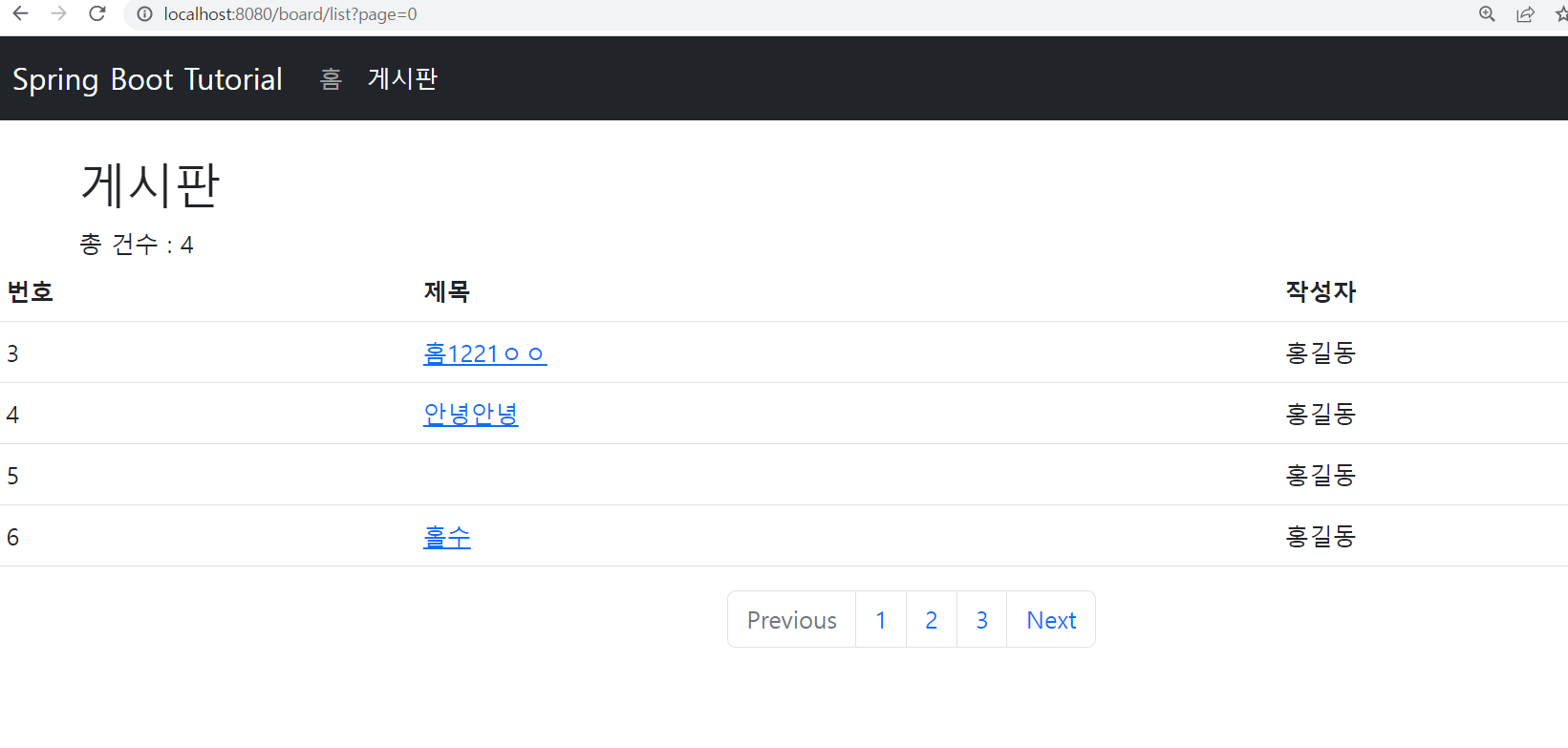
Bootstrap 에서 페이지 UI 복사
https://getbootstrap.com/docs/5.2/components/pagination/#overview
Pagination
Documentation and examples for showing pagination to indicate a series of related content exists across multiple pages.
getbootstrap.com
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item disabled">
<a class="page-link">Previous</a>
</li>
<li class="page-item"><a class="page-link" href="#">1</a></li>
<li class="page-item"><a class="page-link" href="#">2</a></li>
<li class="page-item"><a class="page-link" href="#">3</a></li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
</nav>
페이징 코드 추가
PagingAndSortingRepository<User, Long> repository = // … get access to a bean
Page<User> users = repository.findAll(PageRequest.of(1, 20));
BoardController.java
@GetMapping("/list")
public String list(Model model) {
Page<Board> boards = boardRepository.findAll(PageRequest.of(0, 20));
model.addAttribute("boards", boards);
return "/board/list";
}
list.html
<!doctype html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head th:replace="fragments/common :: head('게시판')">
</head>
<body class="d-flex flex-column h-100">
<header th:replace="fragments/common :: menu('board')">
</header>
<!-- Begin page content -->
<main class="flex-shrink-0">
<div class="container">
<h2>게시판</h2>
<div>총 건수 : <span th:text="${boards.totalElements}"></span></div>
</div>
<table class="table">
<thead>
<tr>
<th scope="col">번호</th>
<th scope="col">제목</th>
<th scope="col">작성자</th>
</tr>
</thead>
<tbody>
<tr th:each="board : ${boards}">
<td th:text="${board.id}">Mark</td>
<td><a th:text="${board.title}" th:href="@{/board/form(id=${board.id})}">Otto</a></td>
<td>홍길동</td>
</tr>
</tbody>
</table>
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item disabled">
<a class="page-link">Previous</a>
</li>
<li class="page-item"><a class="page-link" href="#">1</a></li>
<li class="page-item"><a class="page-link" href="#">2</a></li>
<li class="page-item"><a class="page-link" href="#">3</a></li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
</nav>
<div class="text-end">
<a type="button" class="btn btn-primary" th:href="@{/board/form}">쓰기</a>
</div>
</div>
</main>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa" crossorigin="anonymous"></script>
</body>
</html>
<div class="container">
<h2>게시판</h2>
<div>총 건수 : <span>4</span></div>
</div>
Pageable 인터페이스 사용하기
@GetMapping("/list")
public String list(Model model, Pageable pageable) {
Page<Board> boards = boardRepository.findAll(pageable);
model.addAttribute("boards", boards);
return "/board/list";
}
파라미터로 page=0
파라미터로 page=1
thymeleaf number loop 사용하기
<th:block th:each="i: ${#numbers.sequence(0, response.count - 1)}">
<button th:if="${response.page == i}" class="active">Dummy</button>
<button th:unless="${response.page == i}">Dummy</button>
</th:block>
list.html
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item disabled">
<a class="page-link">Previous</a>
</li>
<li class="page-item" th:each="i: ${#numbers.sequence(startPage, endPage)}"><a class="page-link" href="#" th:text="${i}">1</a></li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
</nav>
BoardController.java
@GetMapping("/list")
public String list(Model model, @PageableDefault(size=2) Pageable pageable) {
Page<Board> boards = boardRepository.findAll(pageable);
int startPage = Math.max(1, boards.getPageable().getPageNumber() - 4);
int endPage = Math.min(boards.getTotalPages(), boards.getPageable().getPageNumber() + 4);
model.addAttribute("startPage", startPage);
model.addAttribute("endPage", endPage);
model.addAttribute("boards", boards);
return "/board/list";
}
@PageableDefault 어노테이션을 이용해 기본 사이즈 값을 2로 설정했다.
th:classappend 를 사용해 현재 페이지 disableld 하기
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == 1} ? 'disabled'">
<a class="page-link" >Previous</a>
</li>
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == i} ? 'disabled'" th:each="i: ${#numbers.sequence(startPage, endPage)}">
<a class="page-link" href="#" th:text="${i}">1</a>
</li>
<li class="page-item" th:classappend="${boards.totalPages == boards.pageable.pageNumber + 1} ? 'disabled'" >
<a class="page-link" href="#">Next</a>
</li>
</ul>
</nav>
previous, next - disabled 추가
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item">
<a class="page-link" th:classappend="${boards.pageable.pageNumber + 1 == 1} ? 'disabled'" >Previous</a>
</li>
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == i} ? 'disabled'" th:each="i: ${#numbers.sequence(startPage, endPage)}"><a class="page-link" href="#" th:text="${i}">1</a></li>
<li class="page-item">
<a class="page-link" th:classappend="${boards.totalPages == boards.pageable.pageNumber + 1} ? 'disabled'" href="#">Next</a>
</li>
</ul>
</nav>
previous, next, number - 링크 추가
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == 1} ? 'disabled'">
<a class="page-link" href="#" th:href="@{/board/list(page=${boards.pageable.pageNumber - 1})}" >Previous</a>
</li>
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == i} ? 'disabled'" th:each="i: ${#numbers.sequence(startPage, endPage)}">
<a class="page-link" href="#" th:href="@{/board/list(page=${i - 1})}" th:text="${i}">1</a>
</li>
<li class="page-item" th:classappend="${boards.totalPages == boards.pageable.pageNumber + 1} ? 'disabled'" >
<a class="page-link" href="#" th:href="@{/board/list(page=${boards.pageable.pageNumber + 1})}">Next</a>
</li>
</ul>
</nav>
페이지 검색 기능 추가
Bootstrap 에서 inline form 코드 복사
https://getbootstrap.com/docs/5.2/forms/layout/#inline-forms
Layout
Give your forms some structure—from inline to horizontal to custom grid implementations—with our form layout options.
getbootstrap.com
<form class="row row-cols-lg-auto g-3 align-items-center">
<div class="col-12">
<label class="visually-hidden" for="inlineFormInputGroupUsername">Username</label>
<div class="input-group">
<div class="input-group-text">@</div>
<input type="text" class="form-control" id="inlineFormInputGroupUsername" placeholder="Username">
</div>
</div>
<div class="col-12">
<label class="visually-hidden" for="inlineFormSelectPref">Preference</label>
<select class="form-select" id="inlineFormSelectPref">
<option selected>Choose...</option>
<option value="1">One</option>
<option value="2">Two</option>
<option value="3">Three</option>
</select>
</div>
<div class="col-12">
<div class="form-check">
<input class="form-check-input" type="checkbox" id="inlineFormCheck">
<label class="form-check-label" for="inlineFormCheck">
Remember me
</label>
</div>
</div>
<div class="col-12">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</form>
<form class="row row-cols-lg-auto g-3 align-items-center">
<div class="col-12">
<div class="input-group">
<label class="visually-hidden" for="searchText">검색</label>
<input type="text" class="form-control" id="searchText" name="searchText">
</div>
</div>
<div class="col-12">
<button type="submit" class="btn btn-primary">검색</button>
</div>
</form>
우측 정렬 하기
https://getbootstrap.com/docs/5.2/utilities/flex/#enable-flex-behaviors
Flex
Quickly manage the layout, alignment, and sizing of grid columns, navigation, components, and more with a full suite of responsive flexbox utilities. For more complex implementations, custom CSS may be necessary.
getbootstrap.com
<div class="d-flex justify-content-end">...</div>
<form class="row row-cols-lg-auto g-3 align-items-center d-flex justify-content-end" >
<div class="col-12">
<div class="input-group">
<label class="visually-hidden" for="searchText">검색</label>
<input type="text" class="form-control" id="searchText" name="searchText">
</div>
</div>
<div class="col-12">
<button type="submit" class="btn btn-primary">검색</button>
</div>
</form>
제목과 내용 검색 후 input 창에 검색 조건 남기기
list.html
<!doctype html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head th:replace="fragments/common :: head('게시판')">
</head>
<body class="d-flex flex-column h-100">
<header th:replace="fragments/common :: menu('board')">
</header>
<!-- Begin page content -->
<main class="flex-shrink-0">
<div class="container">
<h2>게시판</h2>
<div>총 건수 : <span th:text="${boards.totalElements}"></span></div>
<form class="row row-cols-lg-auto g-3 align-items-center d-flex justify-content-end" method="GET" th:action="@{/board/list}">
<div class="col-12">
<div class="input-group">
<label class="visually-hidden" for="searchText">검색</label>
<input type="text" class="form-control" id="searchText" name="searchText" th:value="${param.searchText}">
</div>
</div>
<div class="col-12">
<button type="submit" class="btn btn-outline-secondary">검색</button>
</div>
</form>
<table class="table">
<thead>
<tr>
<th scope="col">번호</th>
<th scope="col">제목</th>
<th scope="col">작성자</th>
</tr>
</thead>
<tbody>
<tr th:each="board : ${boards}">
<td th:text="${board.id}">Mark</td>
<td><a th:text="${board.title}" th:href="@{/board/form(id=${board.id})}">Otto</a></td>
<td>홍길동</td>
</tr>
</tbody>
</table>
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-center">
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == 1} ? 'disabled'">
<a class="page-link" href="#" th:href="@{/board/list(page=${boards.pageable.pageNumber - 1}, searchText=${param.searchText})}" >Previous</a>
</li>
<li class="page-item" th:classappend="${boards.pageable.pageNumber + 1 == i} ? 'disabled'" th:each="i: ${#numbers.sequence(startPage, endPage)}">
<a class="page-link" href="#" th:href="@{/board/list(page=${i - 1}, searchText=${param.searchText})}" th:text="${i}">1</a>
</li>
<li class="page-item" th:classappend="${boards.totalPages == boards.pageable.pageNumber + 1} ? 'disabled'" >
<a class="page-link" href="#" th:href="@{/board/list(page=${boards.pageable.pageNumber + 1}, searchText=${param.searchText})}">Next</a>
</li>
</ul>
</nav>
<div class="text-end">
<a type="button" class="btn btn-primary" th:href="@{/board/form}">쓰기</a>
</div>
</div>
</main>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa" crossorigin="anonymous"></script>
</body>
</html>
form 태그에 method="GET", th:action="" 을 추가해 get방식으로 form 데이터를 전송 한다.
'JPA' 카테고리의 다른 글
JPA - 다대다 관계 한계 극복 (0) | 2022.09.11 |
---|---|
JPA - Spring Security를 이용한 로그인 처리 (0) | 2022.09.10 |
JPA - Jpa를 이용한 RESTful API 만들기 (0) | 2022.09.08 |
JPA - Pageable (0) | 2022.09.02 |
JPA - Paging (0) | 2022.09.01 |