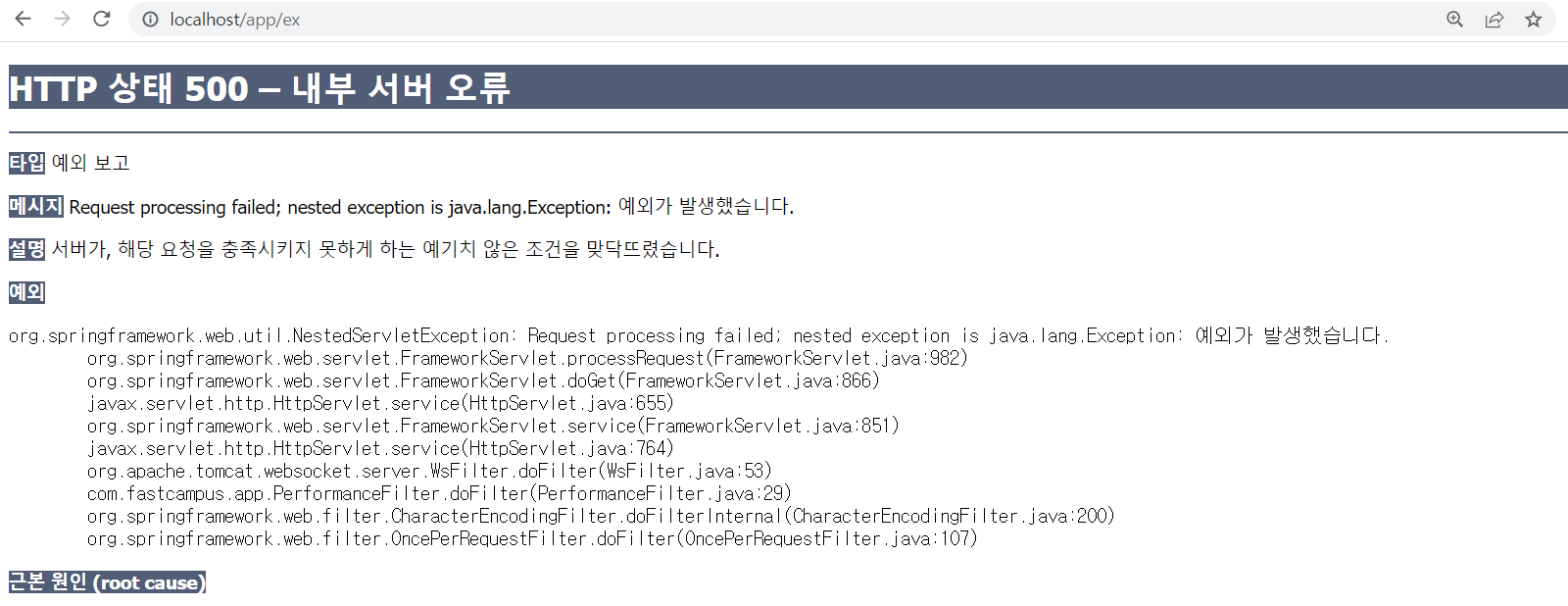
@ResponseStatus
응답 메시지의 상태 코드를 변경할 때 사용
@ControllerAdvice 에 일부로 패키지을 잘 못 입력하자
그러면 500 번 내부서버오류가 날 것이다
GlobalCatcher.java
package com.fastcampus.app;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice("com.fastcampus.bpp")
public class GlobalCatcher {
@ExceptionHandler(Exception.class)
public String catcher(Exception ex, Model m) {
m.addAttribute("ex", ex);
return "error";
}
}
ExceptionController.java
package com.fastcampus.app;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class ExceptionController {
@RequestMapping("/ex")
public String main() throws Exception {
throw new Exception("예외가 발생했습니다.");
}
@RequestMapping("/ex2")
public String main2() throws Exception {
throw new Exception("예외가 발생했습니다.");
}
}
이번에 패키지 주소를 잘 적어서 실행할 때는
200번 코드가 나타나지만 예외 상황이 발생 된 것이므로
상태 코드를 바꾸고 싶다
GlobalCatcher.java
package com.fastcampus.app;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice("com.fastcampus.app")
public class GlobalCatcher {
@ExceptionHandler(Exception.class)
public String catcher(Exception ex, Model m) {
m.addAttribute("ex", ex);
return "error";
}
}
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERRORR) 를 추가해
상태코드를 200 -> 500 으로 바꾸자
package com.fastcampus.app;
import org.springframework.http.HttpStatus;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice("com.fastcampus.app")
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERRORR)
public class GlobalCatcher {
@ExceptionHandler(Exception.class)
public String catcher(Exception ex, Model m) {
m.addAttribute("ex", ex);
return "error";
}
}
isErrorPage 사용
jsp 에서 index에 isErrorPage="true" 추가하면
Controller 에서 model 객체에 예외 값을 넘기지 않아도 사용가능 하다
pageContext 객체를 사용 가능
<%@ page contentType="text/html;charset=utf-8" isErrorPage="true"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>error.jsp</title>
</head>
<body>
<h1>예외가 발생했습니다.</h1>
발생한 예외 : ${ex}<br>
예외 메시지 : ${ex.message}<br>
<ol>
<c:forEach items="${ex.stackTrace}" var="i">
<li>${i.toString()}</li>
</c:forEach>
</ol>
</body>
</html>
<%@ page contentType="text/html;charset=utf-8" isErrorPage="true"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>error.jsp</title>
</head>
<body>
<h1>예외가 발생했습니다.</h1>
<%-- 발생한 예외 : ${ex}<br> --%>
<%-- 예외 메시지 : ${ex.message}<br> --%>
발생한 예외 : ${pageContext.exception}<br>
예외 메시지 : ${pageContext.exception.message}<br>
<ol>
<%-- <c:forEach items="${ex.stackTrace}" var="i"> --%>
<c:forEach items="${pageContext.exception.stackTrace}" var="i">
<li>${i.toString()}</li>
</c:forEach>
</ol>
</body>
</html>
web.xml 에서 error-page 설정
<error-page>
<error-code>400</error-code>
<location>/error400.jsp</location>
</error-page>
<error-page>
<error-code>500</error-code>
<location>/error500.jsp</location>
</error-page>
error400.jsp
<%@ page contentType="text/html;charset=utf-8" isErrorPage="true"%>
[400] 잘 못된 요청입니다
error500.jsp
<%@ page contentType="text/html;charset=utf-8" isErrorPage="true"%>
[500] 서버 장애입니다.
@SimpleMappingExceptionResolver
예외 종류별 뷰 매핑에 사용
sevlet-context.xml 에 등록
<beans:bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver">
<beans:property name="defaultErrorView" value="error"/>
<beans:property name="exceptionMappings">
<beans:props>
<beans:prop key="com.fastcampus.ch2.MyException">error400</beans:prop>
</beans:props>
</beans:property>
<beans:property name="statusCodes">
<beans:props>
<beans:prop key="error400">400</beans:prop>
</beans:props>
</beans:property>
</beans:bean>
상태코드를 400(HttpStatus.BAD_REQUEST)으로 하고 실행해보자
package com.fastcampus.app;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
@ResponseStatus(HttpStatus.BAD_REQUEST)
class MyException extends RuntimeException {
MyException(String msg){
super(msg);
}
MyException(){this("");}
}
@Controller
public class ExceptionController2 {
@RequestMapping("/ex3")
public String main() throws Exception {
throw new MyException("예외가 발생했습니다.");
}
@RequestMapping("/ex4")
public String main2() throws Exception {
throw new Exception("예외가 발생했습니다.");
}
}
400이어야 하는 데 500코드가 발생했다.
이유는 jps 페이지에 isErrorPage="true" 가 상태코드 500인 내부 서버 오류로 나타내기 때문이다
<%@ page contentType="text/html;charset=utf-8" isErrorPage="true"%>
[400] 잘 못된 요청입니다
false로 바꾸자
<%@ page contentType="text/html;charset=utf-8" isErrorPage="false"%>
[400] 잘 못된 요청입니다
ExceptionResolver
예외처리 기본전략
순서대로 처리할 수 있는 지 확인
Maven Dependencied -> spring-webmvc-5.0.7.RELEASE.jar -> org.springframework.web.servlet -> DIspatcherServlet.properties
org.springframework.web.servlet.HandlerExceptionResolver=org.springframework.web.servlet.mvc.method.annotation.ExceptionHandlerExceptionResolver,\
org.springframework.web.servlet.mvc.annotation.ResponseStatusExceptionResolver,\
org.springframework.web.servlet.mvc.support.DefaultHandlerExceptionResolver
정리
1. 컨트롤러 메서드 내에서 try-catch 로 처리
2. 컨트롤러에 @ExceptionHandler 메서드가 처리
3. @ControllerAdvice 클래스의 @ExceptionHandler 메서드가 처리
4. 예외 종류별로 뷰 지정 - SimpleMppingExceptionReslover
5. 응답 상태 코드별로 뷰 지정 - <error-page>
'Spring > Ch2. Spring MVC' 카테고리의 다른 글
Spring MVC - 데이터 변환과 검증 (0) | 2022.09.24 |
---|---|
Spring MVC - DispatcherServlet (0) | 2022.09.23 |
Spring MVC - 예외 처리 (0) | 2022.09.22 |
Spring MVC - 로그인 페이지 만들기3 (1) | 2022.09.21 |
Spring MVC - 로그인 페이지 만들기2 (0) | 2022.09.20 |