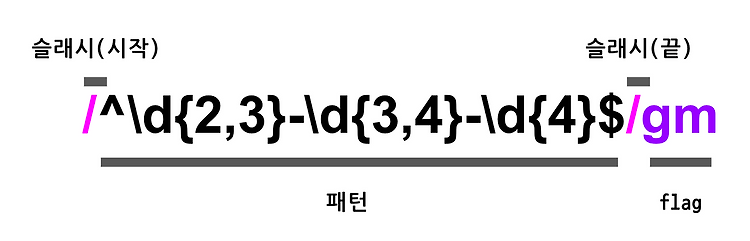
JavaScript - 정규 표현식(Reqular expressions)
2023. 2. 1. 11:32
JavaScript
정규표현식(Regular Expression) 정규식(Regular Expression)은 문자열에서 특정 내용을 찾거나 대체 또는 발췌하는데 사용된다. 정규식 구성 슬래쉬 문자 두개 사이로 정규식 기호가 들어가는 형태이다. 뒤의 i 는 정규식 플래그이다. // 리터럴 방식 const regex = /abc/; // 생성자 방식 const regex = new RegExp("abc"); const regex = new RegExp(/abc/); // 이렇게 해도 됨 정규식 메서드 메서드 의미 ("문자열").match(/정규표현식/플래그) "문자열"에서 "정규표현식"에 매칭되는 항목들을 배열로 반환 ("문자열").replace(/정규표현식/, "대체문자열") "정규표현식"에 매칭되는 항목을 "대체문자열"로..
JavaScript/jQuery - form, input 동적 생성 및 submit
2022. 12. 30. 17:53
JavaScript
Form 태그 역시 document내 엘리먼트이기 때문에 필요할때 문서내에 없더라도 동적으로 Form 태그를 생성하여 action method 등 필요한 속성들을 설정하고 전송할 input 엘리먼트를 form안에 생성하여 submit하는 것이 가능합니다. JavaScript /* Javascript */ // create element (form) var newForm = document.createElement('form'); // set attribute (form) newForm.name = 'newForm'; newForm.method = 'post'; newForm.action = 'https://ifuwanna.tistory.com/196'; newForm.target = '_blank'; /..
jQuery - jQeury를 사용해 쿠키 관리하기(읽기, 생성, 삭제, 생명주기)
2022. 12. 30. 14:19
JavaScript/jQuery
쿠키 쿠키는 클라이언트(브라우저) 로컬에 저장되는 키와 값이 들어있는 작은 데이터 파일입니다. 사용자 인증이 유효한 시간을 명시할 수 있으며, 유효 시간이 정해지면 브라우저가 종료되어도 인증이 유지된다는 특징이 있습니다. [jQuery] 다운로드 및 설치 방법 를 통해 jquery를 다운 받습니다. https://plugins.jquery.com/cookie/ jQuery Cookie 플러그인을 다운 받습니다. 다운로드 받은 js 파일을 페이지에 포함 시킵니다. jquery 플러그인 이므로 jquery 도 있어야 합니다. 쿠키 만들기 // 세션 쿠키 생성 - 브라우저를 닫으면 없으집니다. $.cookie('name', 'value'); // 7일 뒤에 만료되는 쿠키 생성 $.cookie('name', '..
javaScript - Map
2022. 11. 21. 14:26
JavaScript
키, 값 쌍 키 중복 X 순서 O Map Methods Method Description new Map() Creates a new Map set() Sets the value for a key in a Map get() Gets the value for a key in a Map delete() Removes a Map element specified by the key has() Returns true if a key exists in a Map forEach() Calls a function for each key/value pair in a Map entries() Returns an iterator with the [key, value] pairs in a Map size(Property) Re..
javaScript - Set
2022. 11. 21. 14:12
JavaScript
Set Methods 중복 X, 순서 X new Set() Creates a new Set add() Adds a new element to the Set delete() Removes an element from a Set has() Returns true if a value exists in the Set forEach() Invokes a callback for each element in the Set values() Returns an iterator with all the values in a Set size(Property) Returns the number of elements in a Set new Set() const letters = new Set(["a","b","c"]); // C..
JavaScript - Iterables
2022. 11. 21. 14:00
JavaScript
Iterables는 배열과 같은 반복 가능한 객체입니다. Iterables는 간단하고 효율적인 코드로 액세스할 수 있습니다. For Of 문자열 반복 j c y 배열 반복 a b c Set 반복 const letters = new Set(["a","b","c"]); for (const x of letters) { // code block to be executed } a b c Map 반복 apples,500 bananas,300 oranges,200
JavaScript - For In, For of
2022. 11. 17. 10:21
JavaScript
The For In Loop for in statement loops through the properties of an Object: John Doe 25 For In Over Arrays 45 4 9 16 25 Array.forEach() The forEach() method calls a function (a callback function) once for each array element. 45 4 9 16 25 The example above uses only the value parameter const numbers = [45, 4, 9, 16, 25]; let txt = ""; numbers.forEach(myFunction); function myFunction(value) { txt ..
JavaScript - Comparison
2022. 11. 17. 09:53
JavaScript
Comparison Operators == equal to x == 8 false x == 5 true x == "5" true === equal value and equal type x === 5 true x === "5" false != not equal x != 8 true !== not equal value or not equal type x !== 5 false x !== "5" true x !== 8 true > greater than x > 8 false = greater than or equal to x >= 8 false "12" true "2" == "12" false The Nullish Coalescing Operator (??) The ?? operator returns the f..
JavaScript - Booleans
2022. 11. 17. 09:45
JavaScript
Everything Without a "Value" is False The Boolean value of 0 (zero) is false false The Boolean value of -0 (minus zero) is false. false The Boolean value of "" (empty string) is false false The Boolean value of undefined is false false The Boolean value of null is false The Boolean value of NaN is false false Booleans as Objects let x = false; let y = new Boolean(false); // typeof x returns bool..
JavaScript - Math Object
2022. 11. 17. 09:13
JavaScript
The Math Object the Math object has no constructor. The Math object is static. Math.E: 2.718281828459045 Math.PI: 3.141592653589793 Math.SQRT2: 1.4142135623730951 Math.SQRT1_2: 0.7071067811865476 Math.LN2: 0.6931471805599453 Math.LN10: 2.302585092994046 Math.LOG2E: 1.4426950408889634 Math.Log10E: 0.4342944819032518 Number to Integer Math.round(x) Returns x rounded to its nearest integer Math.cei..
JavaScript - Set Date Methods
2022. 11. 17. 08:53
JavaScript
Set Date Methods setDate() Set the day as a number (1-31) setFullYear() Set the year (optionally month and day) setHours() Set the hour (0-23) setMilliseconds() Set the milliseconds (0-999) setMinutes() Set the minutes (0-59) setMonth() Set the month (0-11) setSeconds() Set the seconds (0-59) setTime() Set the time (milliseconds since January 1, 1970) setFullYear() Method sets the year of a date..
JavaScript - Date Formats
2022. 11. 16. 10:45
JavaScript
Date Input ISO Date "2015-03-25" (The International Standard) Short Date "03/25/2015" Long Date "Mar 25 2015" or "25 Mar 2015" Date Get Methods getFullYear() Get year as a four digit number (yyyy) getMonth() Get month as a number (0-11) getDate() Get day as a number (1-31) getDay() Get weekday as a number (0-6) getHours() Get hour (0-23) getMinutes() Get minute (0-59) getSeconds() Get second (0-..
JavaScript - Array Iteration
2022. 11. 15. 16:02
JavaScript
forEach() 각 배열 요소에 대해 한 번씩 함수(콜백 함수)를 호출합니다. 항목 값 아이템 인덱스 어레이 자체 const numbers = [45, 4, 9, 16, 25]; let txt = ""; numbers.forEach(myFunction); function myFunction(value) { txt += value + " "; } map() 이 함수는 3개의 인수를 사용합니다. 항목 값 아이템 인덱스 어레이 자체 콜백 함수가 값 매개변수만 사용하는 경우 인덱스 및 배열 매개변수를 생략할 수 있습니다. 90,8,18,32,50 filter() 테스트를 통과하는 배열 요소로 새 배열을 만듭니다. 45,25 indexOf() 배열에서 요소 값을 검색하고 해당 위치를 반환합니다. Array.in..
JavaScript - Sorting Arrays
2022. 11. 15. 13:58
JavaScript
Sort() Banana,Orange,Apple,Mango Apple,Banana,Mango,Orange reverse() Banana,Orange,Apple,Mango Orange,Mango,Banana,Apple 40,100,1,5,25,10 1,5,10,25,40,100 const points = [40, 100, 1, 5, 25, 10]; points.sort(function(a, b){return b - a}); 40,100,1,5,25,10 100,40,25,10,5,1 비교 기능 function(a, b){return a - b} 함수가 두 값을 비교할 때 sort()값을 비교 함수로 보내고 반환된 값(음수, 0, 양수)에 따라 값을 정렬합니다. 결과가 음수이면 a가 앞에 정렬 b됩니다. 결..
JavaScript - Arrays
2022. 11. 15. 11:27
JavaScript
Looping Array Elements const fruits = ["Banana", "Orange", "Apple", "Mango"]; let fLen = fruits.length; let text = ""; for (let i = 0; i < fLen; i++) { text += "" + fruits[i] + ""; } text += ""; Banana Orange Apple Mango Array.forEach() const fruits = ["Banana", "Orange", "Apple", "Mango"]; let text = ""; fruits.forEach(myFunction); text += ""; function myFunction(value) { text += "" + value + "..
JavaScript - Number Methods
2022. 11. 15. 10:02
JavaScript
Number Methods toString() 숫자를 문자열로 반환 toExponetial() 지수 표기법으로 작성된 숫자를 반환합니다. toFixed() 소수점 이하 자릿수로 작성된 숫자를 반환합니다. toPrecision() 지정된 길이로 작성된 숫자를 반환합니다. ValueOf() 숫자를 숫자로 반환 The toString() Method let x = 123; x.toString(); (123).toString(); (100 + 23).toString(); 123 123 123 The toExponential() Method toExponential()지수 표기법을 사용하여 반올림 및 작성된 숫자로 문자열을 반환합니다. 매개변수는 소수점 뒤의 문자 수를 정의합니다. let x = 9.656; x...
JavaScript - Template Literals
2022. 11. 10. 12:00
JavaScript
Interpolation ${...} Variable Substitutions let firstName = "John"; let lastName = "Doe"; let text = `Welcome ${firstName}, ${lastName}!`; Expression Substitution let price = 10; let VAT = 0.25; let total = `Total: ${(price * (1 + VAT)).toFixed(2)}`; Total: 12.50 HTML Templates let header = "Templates Literals"; let tags = ["template literals", "javascript", "es6"]; let html = `${header}`; for (..
JavaScript - String Search
2022. 11. 10. 11:55
JavaScript
JavaScript Search Methods String indexOf() String lastIndexOf() String search() String match() String matchAll() String includes() String startsWith() String endsWith() indexOf() let str = "Please locate where 'locate' occurs!"; str.indexOf("locate"); 7 lastIndexOf() let text = "Please locate where 'locate' occurs!"; text.lastIndexOf("locate"); 21 indexOf(), lastIndexOf()텍스트가 없으면 -1을 반환합니다. 검색의 ..
JavaScript - String
2022. 11. 10. 11:35
JavaScript
String Length length속성 let text = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let length = text.length; Escape Character Code Result Description \' ' Single quote \" " Double quote \\ \ Backslash Extracting String Parts slice(start, end) substring(start, end) substr(start, length) slice() let text = "Apple, Banana, Kiwi"; let part = text.slice(7, 13); Banana Replacing String Content let text = "Please visit M..
JavaScript - Object
2022. 11. 10. 10:25
JavaScript
객체의 속성에 접근하기 objectName.propertyName or objectName["propertyName"]