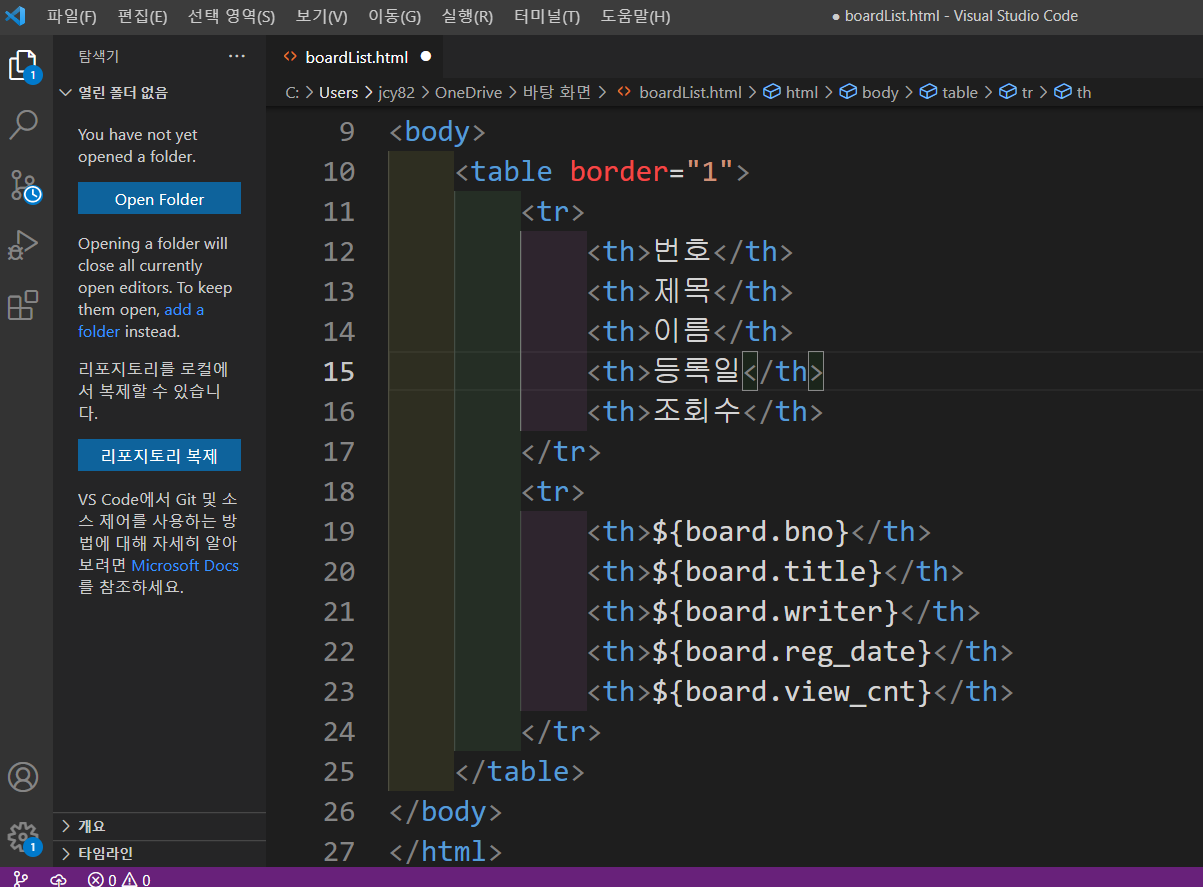
게시판 목록 만들기
boardList.jsp에 게시판 목록 추가
VSCode 를 사용해서 간단하게 작성
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<table border="1">
<tr>
<th>번호</th>
<th>제목</th>
<th>이름</th>
<th>등록일</th>
<th>조회수</th>
</tr>
<c:forEach var="board" items="${list}">
<tr>
<td>${board.bno}</td>
<td>${board.title}</td>
<td>${board.writer}</td>
<td>${board.reg_date}</td>
<td>${board.view_cnt}</td>
</tr>
</c:forEach>
</table>
</div>
</body>
</html>
BoardController.java
package com.jcy.usedhunter.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jcy.usedhunter.domain.BoardDto;
import com.jcy.usedhunter.domain.PageHandler;
import com.jcy.usedhunter.service.BoardService;
@Controller
@RequestMapping("/board")
public class BoardController {
@Autowired
BoardService boardService;
@GetMapping("/list")
public String list(Integer page, Integer pageSize, Model m, HttpServletRequest request) {
if(!loginCheck(request)) {
return "redirect:/login/login?toURL="+request.getRequestURL(); // 로그인을 안했으면 로그인 화면으로 이동
}
if (page==null) {
page=1;
}
if (pageSize==null) {
pageSize=10;
}
try {
int totalCnt = boardService.getCount();
PageHandler ph = new PageHandler(totalCnt, page, pageSize);
Map map = new HashMap();
map.put("offset", (page-1)*pageSize);
map.put("pageSize", pageSize);
List<BoardDto> list = boardService.getPage(map);
m.addAttribute("list", list);
m.addAttribute("ph", ph);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "boardList"; // 로그인을 했으면 게시판 화면으로 이동
}
private boolean loginCheck(HttpServletRequest request) {
// 1. 세션을 얻고
HttpSession session = request.getSession();
// 2. 세션에 id 가 있는 지 확인 있으면 true 를 반환
// if(session.getAttribute("id")!=null) {
// return true;
// } else {
// return false;
// }
return session.getAttribute("id")!=null;
}
}
페이징
PageHandler.java
package com.jcy.usedhunter.domain;
public class PageHandler {
private int totalCnt; // 총 게시물 갯수
private int pageSize; // 한 페이지의 크기
private int naviSize = 10; // 페이지 내비게이션의 크기
private int totalPage; // 전체 페이지의 개수
private int page; // 현재 페이지
private int beginPage; // 내비게이션의 첫번째 페이지
private int endPage; // 내비게이션의 마지막 페이지
private boolean showPrev; // 이전 페이지로 이동하는 링크를 보여줄 것인지의 여부
private boolean showNext; // 다음 페이지로 이동하는 링크를 보여줄 것인지의 여부
public PageHandler(int totalCnt, int page) {
this(totalCnt, page, 10);
}
public PageHandler(int totalCnt, int page, int pageSize) {
this.totalCnt = totalCnt;
this.page = page;
this.pageSize = pageSize;
totalPage = (int)Math.ceil(totalCnt/(double)pageSize);
beginPage = (page-1) / naviSize * 10 + 1;
endPage = Math.min(beginPage + naviSize-1, totalPage);
showPrev = beginPage != 1;
showNext = endPage != totalPage;
}
public void print() {
System.out.println("page = " + page);
System.out.print(showPrev ? "[PREV] " : "");
for(int i = beginPage; i <= endPage; i++) {
System.out.print(i + " ");
}
System.out.println(showNext ? " [NEXT] " : "");
}
public int getTotalCnt() {
return totalCnt;
}
public void setTotalCnt(int totalCnt) {
this.totalCnt = totalCnt;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public int getNaviSize() {
return naviSize;
}
public void setNaviSize(int naviSize) {
this.naviSize = naviSize;
}
public int getTotalPage() {
return totalPage;
}
public void setTotalPage(int totalPage) {
this.totalPage = totalPage;
}
public int getPage() {
return page;
}
public void setPage(int page) {
this.page = page;
}
public int getBeginPage() {
return beginPage;
}
public void setBeginPage(int beginPage) {
this.beginPage = beginPage;
}
public int getEndPage() {
return endPage;
}
public void setEndPage(int endPage) {
this.endPage = endPage;
}
public boolean isShowPrev() {
return showPrev;
}
public void setShowPrev(boolean showPrev) {
this.showPrev = showPrev;
}
public boolean isShowNext() {
return showNext;
}
public void setShowNext(boolean showNext) {
this.showNext = showNext;
}
@Override
public String toString() {
return "PageHandler [totalCnt=" + totalCnt + ", pageSize=" + pageSize + ", naviSize=" + naviSize
+ ", totalPage=" + totalPage + ", page=" + page + ", beginPage=" + beginPage + ", endPage=" + endPage
+ ", showPrev=" + showPrev + ", showNext=" + showNext + "]";
}
}
페이징 테스트
PageHandlerTest.java
package com.jcy.usedhunter;
import static org.junit.Assert.assertTrue;
import org.junit.Test;
import com.jcy.usedhunter.domain.PageHandler;
public class PageHandlerTest {
@Test
public void test() {
PageHandler ph = new PageHandler(250, 1);
ph.print();
assertTrue(ph.getBeginPage()==1);
assertTrue(ph.getEndPage()==10);
}
@Test
public void test2() {
PageHandler ph = new PageHandler(250, 11);
ph.print();
assertTrue(ph.getBeginPage()==11);
assertTrue(ph.getEndPage()==20);
}
@Test
public void test3() {
PageHandler ph = new PageHandler(255, 25);
ph.print();
assertTrue(ph.getBeginPage()==21);
assertTrue(ph.getEndPage()==26);
}
@Test
public void test4() {
PageHandler ph = new PageHandler(255, 10);
ph.print();
assertTrue(ph.getBeginPage()==1);
assertTrue(ph.getEndPage()==10);
}
}
page = 1
1 2 3 4 5 6 7 8 9 10 [NEXT]
page = 11
[PREV] 11 12 13 14 15 16 17 18 19 20 [NEXT]
page = 25
[PREV] 21 22 23 24 25 26
page = 10
1 2 3 4 5 6 7 8 9 10 [NEXT]
boardList.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<table border="1">
<tr>
<th>번호</th>
<th>제목</th>
<th>이름</th>
<th>등록일</th>
<th>조회수</th>
</tr>
<c:forEach var="board" items="${list}">
<tr>
<td>${board.bno}</td>
<td>${board.title}</td>
<td>${board.writer}</td>
<td>${board.reg_date}</td>
<td>${board.view_cnt}</td>
</tr>
</c:forEach>
</table>
<br>
<div>
<c:if test="${ph.showPrev}">
<a href="<c:url value='/board/list?page=${ph.beginPage-1}&pageSize=${ph.pageSize}'/>"><</a>
</c:if>
<c:forEach var="i" begin="${ph.beginPage}" end="${ph.endPage}">
<a href="<c:url value='/board/list?page=${i}&pageSize=${ph.pageSize}'/>">${i}</a>
</c:forEach>
<c:if test="${ph.showNext}">
<a href="<c:url value='/board/list?page=${ph.endPage+1}&pageSize=${ph.pageSize}'/>">></a>
</c:if>
</div>
</div>
</body>
</html>
'프로젝트 > 중고헌터' 카테고리의 다른 글
중고헌터 - 게시판 읽기, 쓰기, 수정, 삭제 구현 2 (0) | 2022.10.06 |
---|---|
중고헌터 - 게시판 읽기, 쓰기, 수정, 삭제 구현 (0) | 2022.10.03 |
중고헌터 - MyBatis로 DAO 작성 (0) | 2022.10.02 |
중고헌터- MyBatis 설정 및 게시판 만들기 준비 (1) | 2022.10.01 |
중고헌터 - DAO(Data Access Object) 작성 2 (0) | 2022.09.29 |