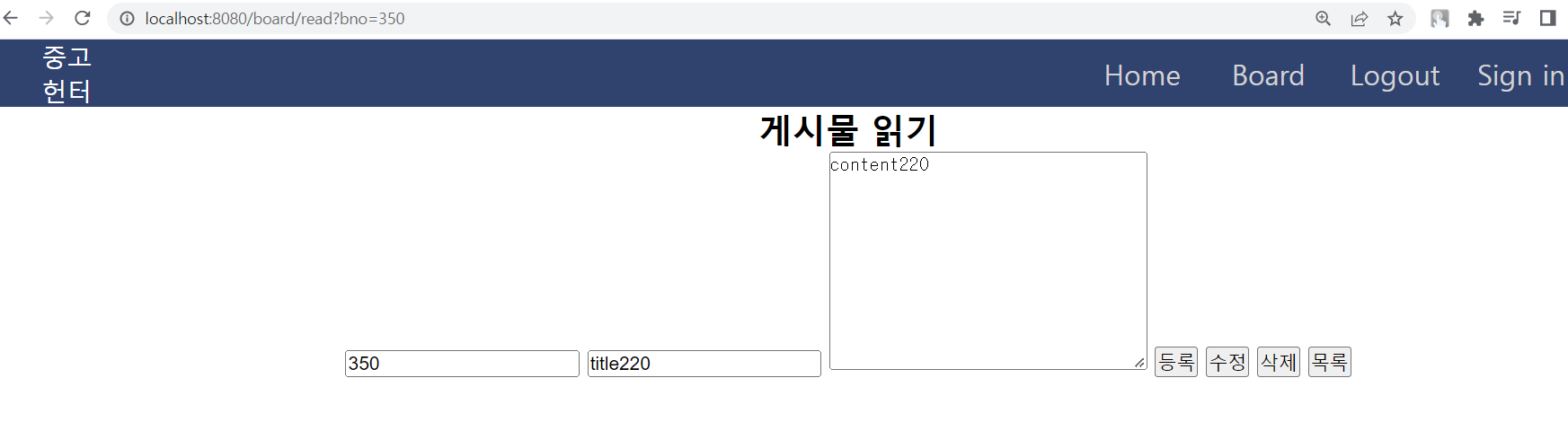
기능별 URI 정의
URL : 리소스 경로, full 경로
URI : identifier, 유일
URL, URL : 비슷함
기능 | URI | HTTP 메서드 | 설명 |
읽기 | /board/read?bno=번호 | GET | 지정된 번호의 게시물을 보여준다. |
삭제 | /board/remove | POST | 게시물을 삭제한다. |
쓰기 | /board/write | GET | 게시물을 작성하기 위한 화면을 보여준다. |
/board/write | POST | 작성한 게시물을 저장한다. | |
수정 | /board/modify?bno=번호 | GET | 게시물을 수정하기 위해 읽어온다. |
/board/modify | POST | 수정된 게시물을 저장한다. |
읽기 기능 구현
BoardController.java
read 메서드 추가
@GetMapping("read")
public String read(Integer bno, Model m) {
try {
BoardDto boardDto = boardService.read(bno);
m.addAttribute(boardDto);
} catch (Exception e) {
e.printStackTrace();
}
return "board";
}
package com.jcy.usedhunter.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jcy.usedhunter.domain.BoardDto;
import com.jcy.usedhunter.domain.PageHandler;
import com.jcy.usedhunter.service.BoardService;
@Controller
@RequestMapping("/board")
public class BoardController {
@Autowired
BoardService boardService;
@GetMapping("/list")
public String list(Integer page, Integer pageSize, Model m, HttpServletRequest request) {
if(!loginCheck(request)) {
return "redirect:/login/login?toURL="+request.getRequestURL(); // 로그인을 안했으면 로그인 화면으로 이동
}
if (page==null) {
page=1;
}
if (pageSize==null) {
pageSize=10;
}
try {
int totalCnt = boardService.getCount();
PageHandler ph = new PageHandler(totalCnt, page, pageSize);
Map map = new HashMap();
map.put("offset", (page-1)*pageSize);
map.put("pageSize", pageSize);
List<BoardDto> list = boardService.getPage(map);
m.addAttribute("list", list);
m.addAttribute("ph", ph);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "boardList"; // 로그인을 했으면 게시판 화면으로 이동
}
@GetMapping("read")
public String read(Integer bno, Model m) {
try {
BoardDto boardDto = boardService.read(bno);
m.addAttribute(boardDto);
} catch (Exception e) {
e.printStackTrace();
}
return "board";
}
private boolean loginCheck(HttpServletRequest request) {
// 1. 세션을 얻고
HttpSession session = request.getSession();
// 2. 세션에 id 가 있는 지 확인 있으면 true 를 반환
// if(session.getAttribute("id")!=null) {
// return true;
// } else {
// return false;
// }
return session.getAttribute("id")!=null;
}
}
board.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<h2>게시물 읽기</h2>
<form action="" id="form">
<input type="text" name="bno" value="${boardDto.bno}" readonly="readonly">
<input type="text" name="title" value="${boardDto.title}" readonly="readonly">
<textarea name="content" id="" cols="30" rows="10" readonly="readonly">${boardDto.content}</textarea>
<button type="button" id="writeBtn" class="btm">등록</button>
<button type="button" id="modifyBtn" class="btm">수정</button>
<button type="button" id="removeBtn" class="btm">삭제</button>
<button type="button" id="listBtn" class="btm">목록</button>
</form>
</div>
</body>
</html>
목록에 제목 클릭해서 읽기 페이지로 이동하기
boardList.jsp
<c:forEach var="boardDto" items="${list}">
<tr>
<td>${boardDto.bno}</td>
<td><a href="<c:url value='/board/read?bno=${boardDto.bno}&page=${page}&pageSize=${pageSize}'/>">${boardDto.title}</a></td>
<td>${boardDto.writer}</td>
<td>${boardDto.reg_date}</td>
<td>${boardDto.view_cnt}</td>
</tr>
</c:forEach>
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<table border="1">
<tr>
<th>번호</th>
<th>제목</th>
<th>이름</th>
<th>등록일</th>
<th>조회수</th>
</tr>
<c:forEach var="boardDto" items="${list}">
<tr>
<td>${boardDto.bno}</td>
<td><a href="<c:url value='/board/read?bno=${boardDto.bno}&page=${page}&pageSize=${pageSize}'/>">${boardDto.title}</a></td>
<td>${boardDto.writer}</td>
<td>${boardDto.reg_date}</td>
<td>${boardDto.view_cnt}</td>
</tr>
</c:forEach>
</table>
<br>
<div>
<c:if test="${ph.showPrev}">
<a href="<c:url value='/board/list?page=${ph.beginPage-1}&pageSize=${ph.pageSize}'/>"><</a>
</c:if>
<c:forEach var="i" begin="${ph.beginPage}" end="${ph.endPage}">
<a href="<c:url value='/board/list?page=${i}&pageSize=${ph.pageSize}'/>">${i}</a>
</c:forEach>
<c:if test="${ph.showNext}">
<a href="<c:url value='/board/list?page=${ph.endPage+1}&pageSize=${ph.pageSize}'/>">></a>
</c:if>
</div>
</div>
</body>
</html>
jQuery를 사용해서 목록 버튼을 눌르면 전 페이지로 이동하기
board.jsp
jQuery 를 cnd 으로 사용하기
<head> 부분에 추가
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
<script>
$(document).ready(function(){ // main
$('#listBtn').on("click", function(){
location.href = "<c:url value='/board/list'/>?page=${page}&pageSize=${pageSize}";
});
});
</script>
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<h2>게시물 읽기</h2>
<form action="" id="form">
<input type="text" name="bno" value="${boardDto.bno}" readonly="readonly">
<input type="text" name="title" value="${boardDto.title}" readonly="readonly">
<textarea name="content" id="" cols="30" rows="10" readonly="readonly">${boardDto.content}</textarea>
<button type="button" id="writeBtn" class="btm">등록</button>
<button type="button" id="modifyBtn" class="btm">수정</button>
<button type="button" id="removeBtn" class="btm">삭제</button>
<button type="button" id="listBtn" class="btm">목록</button>
</form>
</div>
<script>
$(document).ready(function(){ // main
$('#listBtn').on("click", function(){
location.href = "<c:url value='/board/list'/>?page=${page}&pageSize=${pageSizs}";
});
});
</script>
</body>
</html>
삭제 버튼 누르면 삭제 하는 기능
BoardController.java
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m) {
String writer = (String)session.getAttribute("id");
try {
boardService.remove(bno, writer);
} catch (Exception e) {
e.printStackTrace();
}
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
package com.jcy.usedhunter.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jcy.usedhunter.domain.BoardDto;
import com.jcy.usedhunter.domain.PageHandler;
import com.jcy.usedhunter.service.BoardService;
@Controller
@RequestMapping("/board")
public class BoardController {
@Autowired
BoardService boardService;
@GetMapping("/list")
public String list(Integer page, Integer pageSize, Model m, HttpServletRequest request) {
if(!loginCheck(request)) {
return "redirect:/login/login?toURL="+request.getRequestURL(); // 로그인을 안했으면 로그인 화면으로 이동
}
if (page==null) {
page=1;
}
if (pageSize==null) {
pageSize=10;
}
try {
int totalCnt = boardService.getCount();
PageHandler ph = new PageHandler(totalCnt, page, pageSize);
Map map = new HashMap();
map.put("offset", (page-1)*pageSize);
map.put("pageSize", pageSize);
List<BoardDto> list = boardService.getPage(map);
m.addAttribute("list", list);
m.addAttribute("ph", ph);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "boardList"; // 로그인을 했으면 게시판 화면으로 이동
}
@GetMapping("read")
public String read(Integer bno, Integer page, Integer pageSize, Model m) {
try {
BoardDto boardDto = boardService.read(bno);
// m.addAttribute("boardDto", boardDto); 아래와 같은 코드
m.addAttribute(boardDto);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
e.printStackTrace();
}
return "board";
}
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m) {
String writer = (String)session.getAttribute("id");
try {
boardService.remove(bno, writer);
} catch (Exception e) {
e.printStackTrace();
}
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
private boolean loginCheck(HttpServletRequest request) {
// 1. 세션을 얻고
HttpSession session = request.getSession();
// 2. 세션에 id 가 있는 지 확인 있으면 true 를 반환
// if(session.getAttribute("id")!=null) {
// return true;
// } else {
// return false;
// }
return session.getAttribute("id")!=null;
}
}
board.java
<script>
$(document).ready(function(){ // main
$('#listBtn').on("click", function(){
location.href = "<c:url value='/board/list'/>?page=${page}&pageSize=${pageSize}";
});
$('#removeBtn').on("click", function(){
let form = $('#form');
form.attr("action", "<c:url value='/board/remove'/>?page=${page}&pageSize=${pageSize}");
form.attr("method", "post");
form.submit();
});
});
</script>
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<h2>게시물 읽기</h2>
<form action="" id="form">
<input type="text" name="bno" value="${boardDto.bno}" readonly="readonly">
<input type="text" name="title" value="${boardDto.title}" readonly="readonly">
<textarea name="content" id="" cols="30" rows="10" readonly="readonly">${boardDto.content}</textarea>
<button type="button" id="writeBtn" class="btm">등록</button>
<button type="button" id="modifyBtn" class="btm">수정</button>
<button type="button" id="removeBtn" class="btm">삭제</button>
<button type="button" id="listBtn" class="btm">목록</button>
</form>
</div>
<script>
$(document).ready(function(){ // main
$('#listBtn').on("click", function(){
location.href = "<c:url value='/board/list'/>?page=${page}&pageSize=${pageSize}";
});
$('#removeBtn').on("click", function(){
let form = $('#form');
form.attr("action", "<c:url value='/board/remove'/>?page=${page}&pageSize=${pageSize}");
form.attr("method", "post");
form.submit();
});
});
</script>
</body>
</html>
삭제 버튼 누르면 confirm 창 나타나게 하기
if (!confirm("정말로 삭제하시겠습니까?")) return;
<script>
$(document).ready(function(){ // main
$('#listBtn').on("click", function(){
location.href = "<c:url value='/board/list'/>?page=${page}&pageSize=${pageSize}";
});
$('#removeBtn').on("click", function(){
if (!confirm("정말로 삭제하시겠습니까?")) return;
let form = $('#form');
form.attr("action", "<c:url value='/board/remove'/>?page=${page}&pageSize=${pageSize}");
form.attr("method", "post");
form.submit();
});
});
</script>
삭제 완료시 alert 메시지 창 나타내기
BoardController.java
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m) {
String writer = (String)session.getAttribute("id");
try {
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
int rowCnt = boardService.remove(bno, writer);
if(rowCnt == 1) {
m.addAttribute("msg", "DEL_OK");
return "redirect:/board/list";
}
} catch (Exception e) {
e.printStackTrace();
}
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
package com.jcy.usedhunter.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jcy.usedhunter.domain.BoardDto;
import com.jcy.usedhunter.domain.PageHandler;
import com.jcy.usedhunter.service.BoardService;
@Controller
@RequestMapping("/board")
public class BoardController {
@Autowired
BoardService boardService;
@GetMapping("/list")
public String list(Integer page, Integer pageSize, Model m, HttpServletRequest request) {
if(!loginCheck(request)) {
return "redirect:/login/login?toURL="+request.getRequestURL(); // 로그인을 안했으면 로그인 화면으로 이동
}
if (page==null) {
page=1;
}
if (pageSize==null) {
pageSize=10;
}
try {
int totalCnt = boardService.getCount();
PageHandler ph = new PageHandler(totalCnt, page, pageSize);
Map map = new HashMap();
map.put("offset", (page-1)*pageSize);
map.put("pageSize", pageSize);
List<BoardDto> list = boardService.getPage(map);
m.addAttribute("list", list);
m.addAttribute("ph", ph);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "boardList"; // 로그인을 했으면 게시판 화면으로 이동
}
@GetMapping("read")
public String read(Integer bno, Integer page, Integer pageSize, Model m) {
try {
BoardDto boardDto = boardService.read(bno);
// m.addAttribute("boardDto", boardDto); 아래와 같은 코드
m.addAttribute(boardDto);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
e.printStackTrace();
}
return "board";
}
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m) {
String writer = (String)session.getAttribute("id");
try {
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
int rowCnt = boardService.remove(bno, writer);
if(rowCnt == 1) {
m.addAttribute("msg", "DEL_OK");
return "redirect:/board/list";
}
} catch (Exception e) {
e.printStackTrace();
}
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
private boolean loginCheck(HttpServletRequest request) {
// 1. 세션을 얻고
HttpSession session = request.getSession();
// 2. 세션에 id 가 있는 지 확인 있으면 true 를 반환
// if(session.getAttribute("id")!=null) {
// return true;
// } else {
// return false;
// }
return session.getAttribute("id")!=null;
}
}
boardList.jsp
<script>
let msg = "${param.msg}"
if(msg=="DEL_OK") alert("성공적으로 삭제되었습니다.");
</script>
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="loginOutLink" value="${sessionScope.id==null ? '/login/login' : '/login/logout'}"/>
<c:set var="loginOut" value="${sessionScope.id==null ? 'Login' : 'Logout'}"/>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>중고헌터</title>
<link rel="stylesheet" href="<c:url value='/css/menu.css'/>">
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
</head>
<body>
<div id="menu">
<ul>
<li id="logo">중고헌터</li>
<li><a href="<c:url value='/'/>">Home</a></li>
<li><a href="<c:url value='/board/list'/>">Board</a></li>
<li><a href="<c:url value='${loginOutLink}'/>">${loginOut}</a></li>
<li><a href="<c:url value='/register/add'/>">Sign in</a></li>
<li><a href=""><i class="fas fa-search small"></i></a></li>
</ul>
</div>
<div style="text-align:center">
<table border="1">
<tr>
<th>번호</th>
<th>제목</th>
<th>이름</th>
<th>등록일</th>
<th>조회수</th>
</tr>
<c:forEach var="boardDto" items="${list}">
<tr>
<td>${boardDto.bno}</td>
<td><a href="<c:url value='/board/read?bno=${boardDto.bno}&page=${page}&pageSize=${pageSize}'/>">${boardDto.title}</a></td>
<td>${boardDto.writer}</td>
<td>${boardDto.reg_date}</td>
<td>${boardDto.view_cnt}</td>
</tr>
</c:forEach>
</table>
<br>
<div>
<c:if test="${ph.showPrev}">
<a href="<c:url value='/board/list?page=${ph.beginPage-1}&pageSize=${ph.pageSize}'/>"><</a>
</c:if>
<c:forEach var="i" begin="${ph.beginPage}" end="${ph.endPage}">
<a href="<c:url value='/board/list?page=${i}&pageSize=${ph.pageSize}'/>">${i}</a>
</c:forEach>
<c:if test="${ph.showNext}">
<a href="<c:url value='/board/list?page=${ph.endPage+1}&pageSize=${ph.pageSize}'/>">></a>
</c:if>
</div>
</div>
<script>
let msg = "${param.msg}"
if(msg=="DEL_OK") alert("성공적으로 삭제되었습니다.");
</script>
</body>
</html>
삭제 실패 했을 때 alert 창 나타내기
BoardController.java
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m) {
String writer = (String)session.getAttribute("id");
try {
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
int rowCnt = boardService.remove(bno, writer);
if(rowCnt != 1)
throw new Exception("board remove error");
m.addAttribute("msg", "DEL_OK");
} catch (Exception e) {
e.printStackTrace();
m.addAttribute("msg", "DEL_ERROR");
}
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
package com.jcy.usedhunter.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jcy.usedhunter.domain.BoardDto;
import com.jcy.usedhunter.domain.PageHandler;
import com.jcy.usedhunter.service.BoardService;
@Controller
@RequestMapping("/board")
public class BoardController {
@Autowired
BoardService boardService;
@GetMapping("/list")
public String list(Integer page, Integer pageSize, Model m, HttpServletRequest request) {
if(!loginCheck(request)) {
return "redirect:/login/login?toURL="+request.getRequestURL(); // 로그인을 안했으면 로그인 화면으로 이동
}
if (page==null) {
page=1;
}
if (pageSize==null) {
pageSize=10;
}
try {
int totalCnt = boardService.getCount();
PageHandler ph = new PageHandler(totalCnt, page, pageSize);
Map map = new HashMap();
map.put("offset", (page-1)*pageSize);
map.put("pageSize", pageSize);
List<BoardDto> list = boardService.getPage(map);
m.addAttribute("list", list);
m.addAttribute("ph", ph);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "boardList"; // 로그인을 했으면 게시판 화면으로 이동
}
@GetMapping("read")
public String read(Integer bno, Integer page, Integer pageSize, Model m) {
try {
BoardDto boardDto = boardService.read(bno);
// m.addAttribute("boardDto", boardDto); 아래와 같은 코드
m.addAttribute(boardDto);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
e.printStackTrace();
}
return "board";
}
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m) {
String writer = (String)session.getAttribute("id");
try {
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
int rowCnt = boardService.remove(bno, writer);
if(rowCnt != 1)
throw new Exception("board remove error");
m.addAttribute("msg", "DEL_OK");
} catch (Exception e) {
e.printStackTrace();
m.addAttribute("msg", "DEL_ERROR");
}
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
private boolean loginCheck(HttpServletRequest request) {
// 1. 세션을 얻고
HttpSession session = request.getSession();
// 2. 세션에 id 가 있는 지 확인 있으면 true 를 반환
// if(session.getAttribute("id")!=null) {
// return true;
// } else {
// return false;
// }
return session.getAttribute("id")!=null;
}
}
boardList.jsp
<script>
let msg = "${param.msg}"
if(msg=="DEL_OK") alert("성공적으로 삭제되었습니다.");
if(msg=="DEL_ERROR") alert("삭제를 실패했습니다.");
</script>
하지만 파라미터에 DEL_ERROR 가 남아있기 때문에 새로고침 시 계속 삭제 실패 창이 나타난다.
때문에 RedirectAttribute 를 사용하자
BoardController.java
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m, RedirectAttributes rttr) {
String writer = (String)session.getAttribute("id");
try {
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
int rowCnt = boardService.remove(bno, writer);
if(rowCnt != 1)
throw new Exception("board remove error");
rttr.addFlashAttribute("msg", "DEL_OK");
} catch (Exception e) {
e.printStackTrace();
rttr.addFlashAttribute("msg", "DEL_ERROR");
}
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
package com.jcy.usedhunter.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.jcy.usedhunter.domain.BoardDto;
import com.jcy.usedhunter.domain.PageHandler;
import com.jcy.usedhunter.service.BoardService;
@Controller
@RequestMapping("/board")
public class BoardController {
@Autowired
BoardService boardService;
@GetMapping("/list")
public String list(Integer page, Integer pageSize, Model m, HttpServletRequest request) {
if(!loginCheck(request)) {
return "redirect:/login/login?toURL="+request.getRequestURL(); // 로그인을 안했으면 로그인 화면으로 이동
}
if (page==null) {
page=1;
}
if (pageSize==null) {
pageSize=10;
}
try {
int totalCnt = boardService.getCount();
PageHandler ph = new PageHandler(totalCnt, page, pageSize);
Map map = new HashMap();
map.put("offset", (page-1)*pageSize);
map.put("pageSize", pageSize);
List<BoardDto> list = boardService.getPage(map);
m.addAttribute("list", list);
m.addAttribute("ph", ph);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "boardList"; // 로그인을 했으면 게시판 화면으로 이동
}
@GetMapping("read")
public String read(Integer bno, Integer page, Integer pageSize, Model m) {
try {
BoardDto boardDto = boardService.read(bno);
// m.addAttribute("boardDto", boardDto); 아래와 같은 코드
m.addAttribute(boardDto);
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
} catch (Exception e) {
e.printStackTrace();
}
return "board";
}
@PostMapping("remove")
public String remove(HttpSession session, Integer bno, Integer page, Integer pageSize, Model m, RedirectAttributes rttr) {
String writer = (String)session.getAttribute("id");
try {
m.addAttribute("page", page);
m.addAttribute("pageSize", pageSize);
int rowCnt = boardService.remove(bno, writer);
if(rowCnt != 1)
throw new Exception("board remove error");
rttr.addFlashAttribute("msg", "DEL_OK");
} catch (Exception e) {
e.printStackTrace();
rttr.addFlashAttribute("msg", "DEL_ERROR");
}
return "redirect:/board/list"; // 모델에 담으면 "redirect:/board/list?page=&pageSize" 으로 자동으로 생성
}
private boolean loginCheck(HttpServletRequest request) {
// 1. 세션을 얻고
HttpSession session = request.getSession();
// 2. 세션에 id 가 있는 지 확인 있으면 true 를 반환
// if(session.getAttribute("id")!=null) {
// return true;
// } else {
// return false;
// }
return session.getAttribute("id")!=null;
}
}
boardList,jsp
param.msg 를 msg 바꿔주자
<script>
let msg = "${msg}"
if(msg=="DEL_OK") alert("성공적으로 삭제되었습니다.");
if(msg=="DEL_ERROR") alert("삭제를 실패했습니다.");
</script>
'프로젝트 > 중고헌터' 카테고리의 다른 글
중고헌터 - 게시판 검색 기능 구현하기 (1) | 2022.10.06 |
---|---|
중고헌터 - 게시판 읽기, 쓰기, 수정, 삭제 구현 2 (0) | 2022.10.06 |
중고헌터 - 게시판 목록 만들기 (0) | 2022.10.03 |
중고헌터 - MyBatis로 DAO 작성 (0) | 2022.10.02 |
중고헌터- MyBatis 설정 및 게시판 만들기 준비 (1) | 2022.10.01 |